How FujiNet High Score Enabled Games bring a Community Together
FujiNet is more than hardware. I see it as a way to bring people together via a WiFi network adapter. The FujiNet community not only do this by providing ways for people to load software, and make new networkable programs that we all can use, but we provide ways to do things together like competing for high scores in games.
To this end, FujiNet implements a feature called “High Score Enabled” which is a simple way to allow for games to specify where on a disk their high score tables are stored, and to allow for those areas to be written to, even with the game being mounted as read only. When a game marked as High Score Enabled, any writes to the high score sectors cause the FujiNet to temporarily re-mount the disk as read-write, write the resulting sectors, and then close the temporary write, re-mounting again as read-only. The apps.irata.online TNFS server contains a growing number of games which have been adapted to work in this manner.
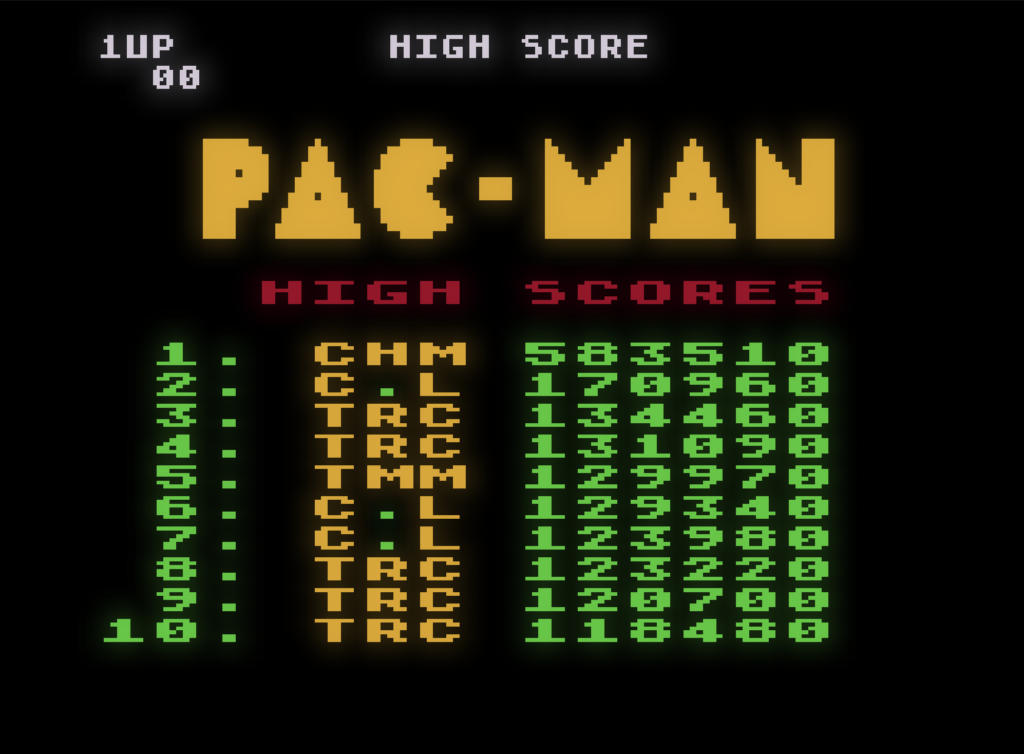
In addition to allowing the read-write of high score tables in these specially marked disk images, there are web pages which get automatically updated with small “scraper” programs, running on the same server and disk as the high-score enabled disk image, which detect the changes in the ATR disk image, and generate a new web page for public viewing, you can see the scores from apps.irata.online‘s High Score Enabled games via scores.irata.online.
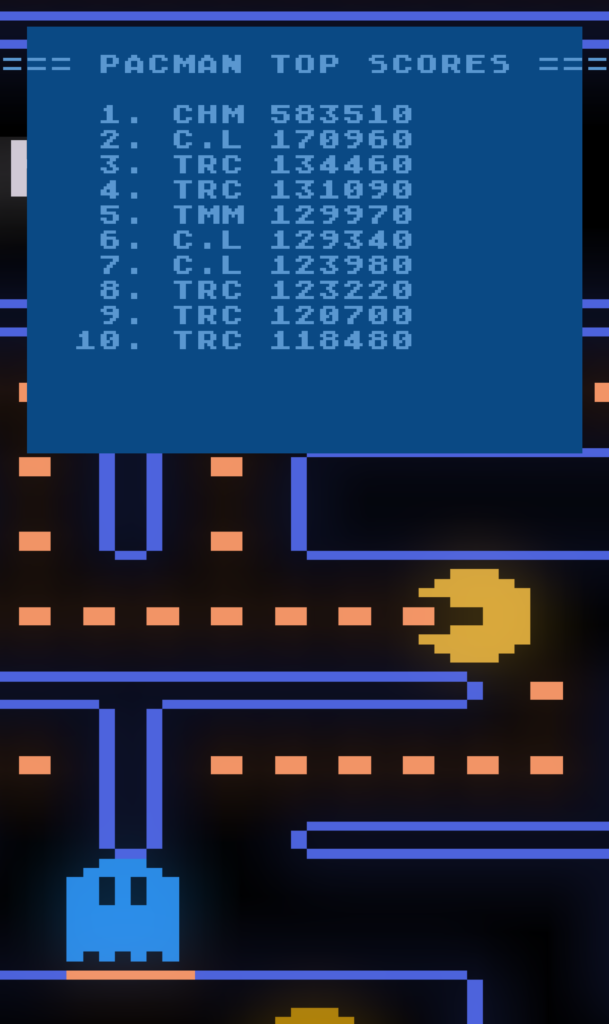
How can I make my game High Score Enabled?
Making your game High Score Enabled can be as simple as:
- Store your high scores on the disk image.
- Alter the ATR header to mark the sectors occupied by high score as writable.
- Implement a scraper if you want to make a web page (optional).
Storing High Scores
This is entirely up to you, as to how you implement it, FujiNet does not care. In the four dozen games that I’ve ported thus far, I have put their implementation in three categories:
- Games that already have a high score table, and save them to disk. Not much to do here but to find where the high score table is, and mark it in the ATR header. Examples of this are Jumpman, Gorf, and Spelunker.
- Games that have a High Score table, but do not save it to disk. We just needed to add routines to write the high score to a disk sector, and to read it back again. An example of this is Baja Buggies.
- Games that do not have a high score table at all. Examples of this are PAC-MAN, Defender, Dig-Dug, and Pengo.
Altering the ATR Header
The initial 16-byte ATR header has a few unused bytes which we are using to hold the High Score Enabled information. They are very simple:
Offset | Size | Description |
+12 | 1 | The number of sectors of the high score table. |
+13 | 2 | The starting sector number (0-65535) of the high score table. |
In fact, the following C program can easily adapt any ATR file:
/**
* Set high-score-mode bytes in ATR file
*/
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int high_score_enable(char *atr, unsigned short start, unsigned char len)
{
FILE *f = fopen(atr,"r+");
unsigned char id[2];
if (!f)
{
perror("error opening file.");
return 1;
}
fread(&id,sizeof(unsigned char),2,f);
if ((id[0] != 0x96) || (id[1] != 0x02))
{
printf("Not a valid ATR file. Aborting.\n");
fclose(f);
return 1;
}
fseek(f,0x0C,SEEK_SET);
fwrite(&len,sizeof(unsigned char),1,f);
fwrite(&start,sizeof(unsigned short),1,f);
fclose(f);
return 0;
}
int main(int argc, char* argv[])
{
if (argc < 4)
{
printf("%s <file.atr> <starting sector> <number of sectors>\n",argv[0]);
return 1;
}
return high_score_enable(argv[1],atoi(argv[2]),atoi(argv[3]));
}
Writing to the Disk: Baja Buggies
Baja Buggies already had a functional high score routine, but it did not write it to disk. The solution to this was to patch Baja Buggies to add two routines to read the high score sectors to and from disk.
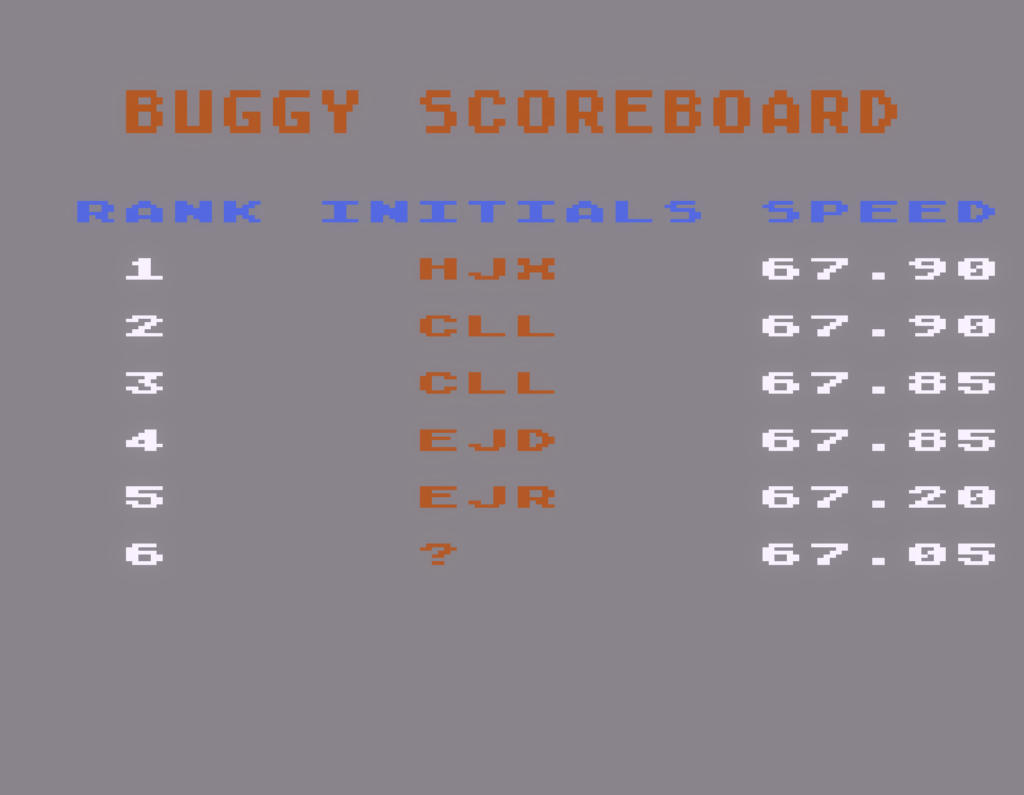
The most straightforward way to do this, is to find the location where the high scores are being displayed from main memory, and to use the operating system SIOV routines to write them to disk. Dumping the display list of the high score screen using Altirra, we see the following:
Altirra> .dumpdlist
37BA: x4 blank 8
37BE: mode 7 @ 37D7
37C1: x2 blank 8
37C3: mode 6
37C4: blank 8
37C5: mode 6
37C6: blank 8
37C7: mode 6
37C8: blank 8
37C9: mode 6
37CA: blank 8
37CB: mode 6
37CC: blank 8
37CD: mode 6
37CE: blank 8
37CF: mode 6
37D0: blank 8
37D1: blank 4
37D2: x2 blank 1
37D4: waitvbl 37BA
So display memory starts at 37D7. Let’s dig in a bit, and see where the high score data starts:
Altirra> dbi 37d7
37D7: 00 00 62 75 67 67 79 00 73 63 6F 72 65 62 6F 61 | buggy scoreboa|
37E7: 72 64 00 00 00 B2 A1 AE AB 00 A9 AE A9 B4 A9 A1 |rd .... ......|
37F7: AC B3 00 B3 B0 A5 A5 A4 00 00 11 00 00 00 00 00 |.. ..... 1 |
3807: 68 6A 78 00 00 00 00 16 17 0E 19 10 00 00 12 00 |hjx 67.90 2 |
3817: 00 00 00 00 63 6C 6C 00 00 00 00 16 17 0E 19 10 | cll 67.90|
3827: 00 00 13 00 00 00 00 00 63 6C 6C 00 00 00 00 16 | 3 cll 6|
3837: 17 0E 18 15 00 00 14 00 00 00 00 00 65 6A 64 00 |7.85 4 ejd |
3847: 00 00 00 16 17 0E 18 15 00 00 15 00 00 00 00 00 | 67.85 5 |
Altirra> dbi 3857
3857: 65 6A 72 00 00 00 00 16 17 0E 12 10 00 00 16 00 |ejr 67.20 6 |
3867: 00 00 00 00 5F 00 00 00 00 00 00 16 17 0E 10 15 | . 67.05|
3877: 00 00 00 B5 B3 A5 00 AB A5 B9 A2 AF A1 B2 A4 00 | ... ........ |
3887: B4 AF 00 00 00 00 A5 AE B4 A5 B2 00 13 00 A9 AE |.. ..... 3 ..|
3897: A9 B4 A9 A1 AC B3 00 00 A9 E0 8D F4 02 A9 08 8D |...... ....".(.|
38A7: C8 02 A9 36 8D C5 02 A9 86 8D C6 02 A9 0E 8D C4 |.".V.."...."....|
38B7: 02 A9 BA 8D 30 02 A9 37 8D 31 02 A9 22 8D 2F 02 |"...P".W.Q".B.O"|
38C7: A9 00 8D 1D D0 8D 03 D2 A9 03 8D 1D 02 8D 1C 02 |. .=..#..#.=".<"|
We see the High Score data starting rather cleanly at $3780, and continuing on a bit past $3880. This means that we need three 128 byte sectors to hold the high score data.
But where do we store it?
Tracing how Baja Buggies loads, we can see that the high score data gets loaded in as part of the boot process:
Altirra> .tracesio on
SIO call tracing is now on.
...
SIO: Device $31[1], command $52, buffer $3780, length $0080, aux $0088 timeout 7.5s | Disk: Read sector
SIO: Device $31[1], command $52, buffer $3800, length $0080, aux $0089 timeout 7.5s | Disk: Read sector
SIO: Device $31[1], command $52, buffer $3880, length $0080, aux $008A timeout 7.5s | Disk: Read sector
...
Tracing the read, we see that the three areas in memory that are occupied by the high score are on sectors $88, $89, and $8A. We just need to write a set of routines which can read and write those sectors, and patch them into the game ATR.
As it turns out, there is some space below the scratch area that Baja Buggies uses at $0489 which is large enough to hold a read and write routine. The following bit of assembler is all that is needed to read and write the high scores to and from disk:
;;
;; Write high scores to disk
;;
OPT h-
DDEVIC = $0300 ;peripheral bus ID number
DUNIT = $0301 ;unit number
DCOMND = $0302 ;bus command ordinal
DSTATS = $0303 ;command type/status return
DBUFLO = $0304 ;data buffer pointer
DBUFHI = $0305
DTIMLO = $0306 ;device timeout in seconds
DBYTLO = $0308 ;number of bytes transferred
DBYTHI = $0309
DAUX1 = $030A ;command auxiliary bytes
DAUX2 = $030B
SIOV = $E459 ; SIO Vector
ORG $0489
LDA #$00 ; We need to put the bottom of the display back to blank
STA $37D2 ; because we are writing it back to disk.
STA $37D3 ; otherwise all hell breaks loose.
LDA #$31 ; Drive 1
STA DDEVIC
LDA #$01 ; Unit 1 (D1:)
STA DUNIT
LDA #'W' ; Write
STA DCOMND
LDA #$80 ; ->Drive
STA DSTATS
LDA #$80 ; $3780
STA DBUFLO
LDA #$37
STA DBUFHI
LDA #$80 ; 128 bytes
STA DBYTLO
LDA #$00
STA DBYTHI
LDA #$88 ; Sector $88
STA DAUX1
LDA #$00
JSR SIOV ; Do it
LDA #$80
STA DSTATS
LDA #$00 ; $3800
STA DBUFLO
LDA #$38
STA DBUFHI
LDA #$89 ; Sector $89
STA DAUX1
JSR SIOV ; do it
LDA #$80
STA DSTATS
LDA #$80 ; $3880
STA DBUFLO
LDA #$38
STA DBUFHI
LDA #$8A ; Sector $8A
STA DAUX1
JSR SIOV ; do it.
JMP $38DF ; Finish and back to Attract mode.
Spending some time in the debugger, we find the attract mode at $38DF, and we find a jump to location $0506 in sector 1 where we can place our additional code. So we assemble the above routine into a bin file (headerless), and write the following bit of C code to inject the code into the unused portion of the disk sector, where it can be called after the game is loaded:
/**
* Patch Baja Buggies to add score code.
* @author Thomas Cherryhomes
* @email thom dot cherryhomes at gmail dot com
* @license gpl v. 3
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SEEK_POS 0x19 /* Right after JMP $0506 in Sector 1 */
#define JUMP_POS 0x45EC /* Replacing the 38DF */
#define MAX_PATCH_SIZE 115
char sectorBuf[MAX_PATCH_SIZE];
const char jump[2]={0x89,0x04};
int main(int argc, char *argv[])
{
FILE *afp; FILE *bfp;
size_t len;
if (argc<3)
{
printf("%s <atr-file> <bin-file>\n",argv[0]);
return 1;
}
afp = fopen(argv[1],"r+");
if (!afp)
{
perror("Could not open ATR file");
return 1; // Bail.
}
bfp = fopen(argv[2],"r");
if (!bfp)
{
perror("Could not open BIN file");
fclose(afp);
return 1; // Bail.
}
if (fseek(afp,SEEK_POS,SEEK_SET))
{
perror("Could not seek to ATR sector");
fclose(afp);
fclose(bfp);
return 1;
}
len = fread(§orBuf[0],sizeof(char),sizeof(sectorBuf),bfp);
fclose(bfp);
fwrite(§orBuf[0],sizeof(char),MAX_PATCH_SIZE,afp);
// Patch 0x45EC to jump to our new routine
fseek(afp,JUMP_POS,SEEK_SET);
fwrite(&jump[0],sizeof(const char),sizeof(jump),afp);
fclose(afp);
// Done.
return 0;
}
Implementing a High Score Table: Kid Grid
Games like Kid Grid, PAC-MAN, Defender, Donkey Kong, and many others do not have any high score capability in them, and need to be patched. Using everything we’ve covered thus far, we’ll add a routine to deal with entering high scores to add this to Kid Grid.
We start by getting the initial game in XEX format, putting it into a form which can be disassembled, disassemble the game, add the high score routine, and assemble the result into a form which can be booted on an ATR disk, with the high score taking up the last two sectors of the disk, 719 and 720.
To get the initial game, we use the Homesoft version of Kid Grid, which can be retrieved from apps.irata.online/Atari_8-bit/Games/Homesoft/K/Kid Grid.xex, and run it directly in Altirra. This has the effect of showing the individual binary segments:
EXE: Loading program 0006-0020 to 2020-203A
EXE: Loading program 0025-0025 to 0244-0244
EXE: Loading program 002A-003B to 2300-2311
EXE: Loading program 0040-0041 to 02E2-02E3
EXE: Jumping to 2300
EXE: Loading program 0046-0270 to 2300-252A
EXE: Loading program 0275-052F to 2533-27ED
EXE: Loading program 0534-0540 to 27F3-27FF
EXE: Loading program 0545-095D to 2827-2C3F
EXE: Loading program 0962-097D to 2C46-2C61
EXE: Loading program 0982-099D to 2C6E-2C89
EXE: Loading program 09A2-09BB to 2C97-2CB0
EXE: Loading program 09C0-09DA to 2CBF-2CD9
EXE: Loading program 09DF-09F9 to 2CE7-2D01
EXE: Loading program 09FE-0A05 to 2D18-2D1F
EXE: Loading program 0A0A-0A18 to 2D64-2D72
EXE: Loading program 0A1D-0A2B to 2D8C-2D9A
EXE: Loading program 0A30-0A3F to 2DB4-2DC3
EXE: Loading program 0A44-0A53 to 2DDC-2DEB
EXE: Loading program 0A58-0A67 to 2E04-2E13
EXE: Loading program 0A6C-0A81 to 2E51-2E66
EXE: Loading program 0A86-0A87 to 2E79-2E7A
EXE: Loading program 0A8C-0A9A to 2E80-2E8E
EXE: Loading program 0A9F-0AB5 to 2EA1-2EB7
EXE: Loading program 0ABA-0AD0 to 2EC9-2EDF
EXE: Loading program 0AD5-0AEB to 2EF1-2F07
EXE: Loading program 0AF0-0B02 to 2F43-2F55
EXE: Loading program 0B07-0B15 to 2F6D-2F7B
EXE: Loading program 0B1A-0B3A to 2FB3-2FD3
EXE: Loading program 0B3F-0B95 to 3000-3056
EXE: Loading program 0B9A-0C00 to 305D-30C3
EXE: Loading program 0C05-0C6A to 30CC-3131
EXE: Loading program 0C6F-0CD6 to 3137-319E
EXE: Loading program 0CDB-1B2E to 31A4-3FF7
EXE: Loading program 1B33-1B34 to 02E2-02E3
EXE: Jumping to 2B00
The Homesoft version does a lot of small loads (as part of its compressed format), which will get in the way of us actually patching the program, so we need to let the loader do its thing, and save the resulting memory image. Again, I use Altirra to do this:
Altirra> .writemem kidgrid.bin 2000 L2000
Wrote 2000-3FFF to kidgrid.bin
The resulting binary can be loaded into the excellent dis6502 tool as a raw binary image loaded at $2000, and the resulting disassembly saved.
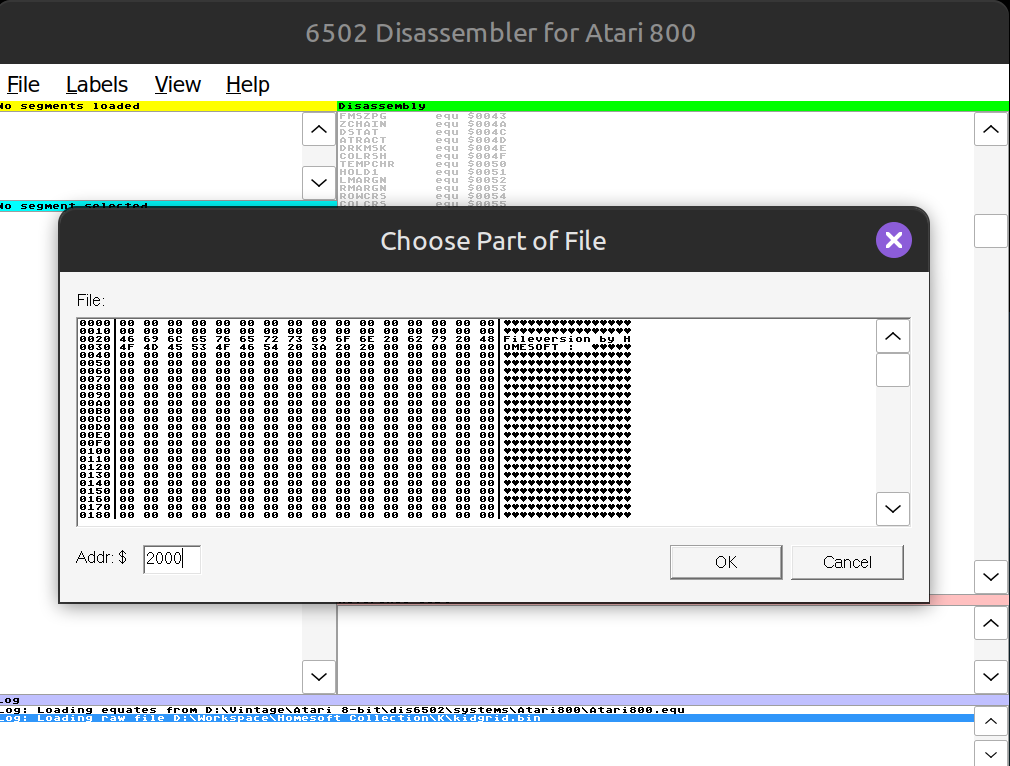
We need to make two modifications to the resulting assembly, to add an ORG and a run address:
;
; Start of code
;
org $2000
; ...
; ... at the bottom...
;
icl "hiscore.asm"
org $02E0
.word $2B00
And we add a new assembly file called hiscore.asm, containing our high score routine. I will not post it here, but you can thumb through it at your leisure. It contains the routines to read and write the high score table to disk, as well as calculate if and where to place the score on the board, and read characters from the keyboard.
Note: Many games, will either completely re-vector the various routines for the SIO, vertical blank, and keyboard interrupts. These interrupts need to be restored when this routine is running, and restored back to their game values when the game resumes.
If you read the README.md for kid-grid, you’ll see that the high score was found at location $0480 in memory, stored high nibble for each digit, so the resulting data for 999999 points is:
90 90 90 90 90 90
Other README.md files in the atari-game-ports directory show what needed to be found and changed as part of the reverse engineering process for each game. Some are still a work in progress that could use some help.
For Kid Grid, I opted to place the high score board in the middle of the screen, using mode 6 characters (20 characters per line, 4 possible colors, and one background), and an altered display list is presented to show this:
hiscore_dlist:
dta $70, $70, $70
dta $4E, $10, $05
dta $0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e
dta $46, .lo(hiscore_txt), .hi(hiscore_txt)
dta $06, $06, $06, $06, $06, $06, $06, $06, $06, $06, $06, $06, $06
dta $4E, $D0, $1C
;dta $4E, $B8, $16
dta $0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e
dta $4E, $00, $20, $0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e,$0e
dta $41, .lo(hiscore_dlist), .hi(hiscore_dlist)
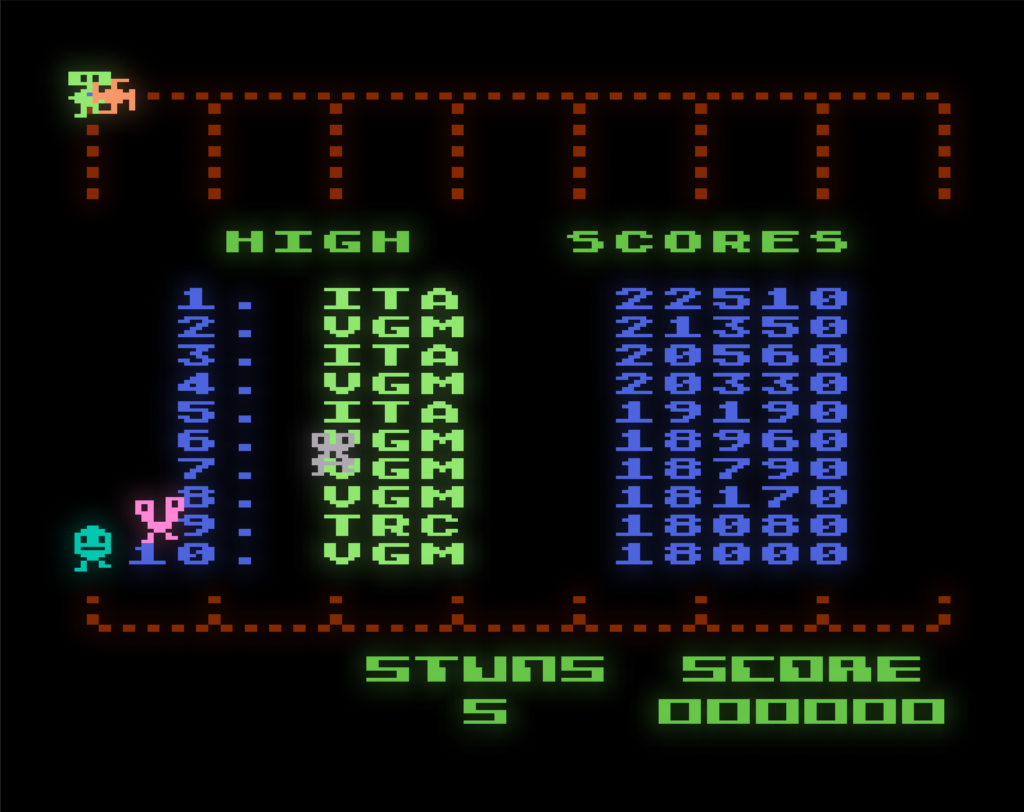
For the high score screen area, space is reserved, which is filled with the data from the two high score sectors, 719, and 720:
hiscore_txt:
.sb ' '
.sb ' high scores '
.sb ' '
HISTR: .ds 128
HISTR2: .ds 128
The actual high score table is assembled separately, without a binary header, and assembles exactly to 256 bytes, so that it can fit within two disk sectors.
;; The High score table. Will be assembled sans header
;; to be written to disk using write-high-score.c
opt h-
HISTR: .SB " 1. "
.SB " 2. "
.SB " 3. "
.SB " 4. "
.SB " 5. "
.SB " 6. "
.SB " 7. "
.SB " 8. "
.SB " 9. "
.SB " 10. "
.SB " "
.SB " "
.SB " "
We also need to write a small tool called write-high-score to place the above data onto the last two sectors of the disk:
/**
* write-high-score - Takes <binfile> and writes to sector 720 of <atr>
*
* @author: Thomas Cherryhomes
* @email: thom dot cherryhomes at gmail dot com
* @license: gpl v. 3
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SEEK_POS 0x16710 // sector 0x2CF
char sectorBuf[256];
int main(int argc, char *argv[])
{
FILE *afp; FILE *bfp;
size_t len;
if (argc<3)
{
printf("%s <atr-file> <bin-file>\n",argv[0]);
return 1;
}
afp = fopen(argv[1],"r+");
if (!afp)
{
perror("Could not open ATR file");
return 1; // Bail.
}
bfp = fopen(argv[2],"r");
if (!bfp)
{
perror("Could not open BIN file");
fclose(afp);
return 1; // Bail.
}
if (fseek(afp,SEEK_POS,SEEK_SET))
{
perror("Could not seek to ATR sector");
fclose(afp);
fclose(bfp);
return 1;
}
len = fread(§orBuf[0],sizeof(char),sizeof(sectorBuf),bfp);
if (len != sizeof(sectorBuf))
{
perror("Could not read BIN file");
fclose(afp);
fclose(bfp);
return 1;
}
fclose(bfp);
fwrite(§orBuf[0],sizeof(char),sizeof(sectorBuf),afp);
fclose(afp);
// Done.
return 0;
}
To make this a bootable ATR, we use the dir2atr utility that’s part of Hias’ AtariSIO tools. We use this, along with a copy of picoboot.bin to create an ATR which contains the game, and a boot sector to load it. The write-high-score tool is used to then write the two high score sectors to disk, using the following Makefile:
AS=mads
CP=cp
SRC=kid_grid.asm
XEX=AUTORUN
LST=kid_grid.lst
ATR="Kid Grid.atr"
BUILD=build
MKDIR=mkdir
DIR2ATR=dir2atr
WRITE_HIGH_SCORE=./write-high-score
HISCORE_TABLE_ASM=hiscore_table.asm
HISCORE_TABLE_BIN=hiscore_table.bin
HIGH_SCORE_ENABLE=./high-score-enable
BOOT_PROGRAM=picoboot.bin
.PHONY: clean pre
all: clean pre xex dist hiscore hiscore_enable
pre:
$(RM) -rf $(BUILD)
$(MKDIR) -p $(BUILD)
xex:
$(AS) $(SRC) -o:$(BUILD)/$(XEX) -l:$(LST)
dist:
$(DIR2ATR) -B $(BOOT_PROGRAM) -S $(ATR) $(BUILD)
hiscore:
$(AS) $(HISCORE_TABLE_ASM) -o:$(BUILD)/$(HISCORE_TABLE_BIN)
$(CC) -o$(WRITE_HIGH_SCORE) $(WRITE_HIGH_SCORE).c
$(WRITE_HIGH_SCORE) $(ATR) $(BUILD)/$(HISCORE_TABLE_BIN)
hiscore_enable:
$(CC) -o$(HIGH_SCORE_ENABLE) $(HIGH_SCORE_ENABLE).c
$(HIGH_SCORE_ENABLE) $(ATR) 719 2
clean:
$(RM) -rf $(BUILD)
$(RM) -rf $(ATR)
$(RM) -rf $(WRITE_HIGH_SCORE)
$(RM) -rf $(HIGH_SCORE_ENABLE)
$(RM) -rf $(LST)
tnfs:
cp kid_grid.atr ~/tnfs
The Score Scraper – Creating the HTML page.
For each of these games, the high score format is determined or created as needed, and because of this, the same data residing in each disk sector can be scraped and reformatted to be displayed as part of an HTML page. Because the scraper and the TNFS server are running on the same machine, each game’s disk image ATR can be monitored independently using the inotify system calls in Linux to detect when the disk image changes, and the resulting HTML page can be re-built.
Kid Grid’s scraper is implemented using the following C code:
/**
* Grab high score from Kid Grid, write to HTML
*
* Linux required. (uses inotify)
*
* @author Thomas Cherryhomes
* @email thom dot cherryhomes at gmail dot com
* @license gpl v. 3
*/
#include <stdio.h>
#include <stdint.h>
#include <stdbool.h>
#include <string.h>
#include <errno.h>
#include <sys/types.h>
#include <sys/inotify.h>
#include <signal.h>
#include <unistd.h>
#include <fcntl.h>
#include <linux/limits.h>
#define EVENT_SIZE ( sizeof(struct inotify_event) )
#define EVENT_BUF_LEN ( 1024 * ( EVENT_SIZE + NAME_MAX ) )
#define PACMAN_SEEK_POS (0x16710)
#define LINE_WIDTH 20
static volatile bool ctrlc = false;
int inotify_fd, inotify_wd;
int inotify_event_len;
char event_buffer[EVENT_BUF_LEN];
void setctrlc(int dummy)
{
ctrlc = true;
}
void kidgrid(char *atr, char *html)
{
unsigned char buf[256];
FILE *fa, *fh;
int i, offset;
printf("Writing new kidgrid.html\n");
fa = fopen(atr,"rb");
fh = fopen(html,"w");
fseek(fa,PACMAN_SEEK_POS,SEEK_SET);
fread(buf,sizeof(unsigned char),sizeof(buf),fa);
/* Process text */
for (i=0;i<sizeof(buf);i++)
{
/* Do very simple ANTIC screen code conversion to ASCII */
unsigned char c = buf[i];
if (c>0x89 && c<0x9A)
c -= 0x80;
if (c>127)
c -= 0xA0;
else if (c<64)
c+=32;
else if (c>64)
c-=32;
buf[i]=c;
}
/* small fix, erase first char in buf */
buf[0]=0x20;
/* start html */
fprintf(fh,"<!DOCTYPE html PUBLIC \"-//W3C//DTD XHTML 1.0 Strict//EN\" \"http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd\">\n");
fprintf(fh,"<html xmlns=\"http://www.w3.org/1999/xhtml\" xml:lang=\"en\" lang=\"en\">\n");
fprintf(fh," <head>\n");
fprintf(fh," <title>Latest Kid Grid High Scores</title>\n");
fprintf(fh," <meta http-equiv=\"content-type\" content=\"text/html; charset=utf-8\" />\n");
fprintf(fh," <meta http-equiv=\"refresh\" content=\"30\" />");
fprintf(fh," <meta name=\"keywords\" content=\" \" />\n");
fprintf(fh," <meta name=\"description\" content=\" \" />\n");
fprintf(fh," <link rel=\"stylesheet\" type=\"text/css\" href=\"kidgrid.css\" media=\"screen\" />\n");
fprintf(fh," <link rel=\"icon\" type=\"image/png\" href=\"icon.png\" />\n");
fprintf(fh," </head>\n");
fprintf(fh," <body>\n");
fprintf(fh," <pre>\n");
/* start body */
offset=0; /* buffer start for hi scores */
fprintf(fh,"\n== KID GRID TOP SCORES ==\n");
for (i=0;i<sizeof(buf);i++)
{
fprintf(fh, "%c", buf[offset++]);
if ((i % LINE_WIDTH) == 0)
fprintf(fh, "\n ");
}
/* end body */
fprintf(fh,"\n");
fprintf(fh," </pre>\n");
fprintf(fh," </body>\n");
fprintf(fh,"</html>\n");
fclose(fh);
fclose(fa);
}
int main(int argc, char *argv[])
{
if (argc < 3)
{
printf("%s <path-to-kidgrid-atr> <path-to-output-html>\n",argv[0]);
return 1;
}
kidgrid(argv[1],argv[2]);
signal(SIGINT, setctrlc);
signal(SIGTERM, setctrlc);
inotify_fd = inotify_init();
if (inotify_fd < 0)
{
perror("inotify_init");
return 1;
}
inotify_wd = inotify_add_watch(inotify_fd, argv[1], IN_MODIFY);
if (inotify_wd == -1)
{
perror("inotify_add_watch");
goto bye2;
}
/* Set for non-blocking */
fcntl (inotify_fd, F_SETFL, fcntl (inotify_fd, F_GETFL) | O_NONBLOCK);
while (!ctrlc)
{
int i;
inotify_event_len = read(inotify_fd, event_buffer, EVENT_BUF_LEN);
i=0;
if (inotify_event_len < 0)
{
usleep(100000);
continue;
}
while (i < inotify_event_len)
{
struct inotify_event *event = ( struct inotify_event * ) &event_buffer[ i ];
kidgrid(argv[1],argv[2]);
i += EVENT_SIZE + event->len;
}
}
/* ctrl-C or termination, close it off. */
printf("Exiting %s\n",argv[0]);
bye:
inotify_rm_watch(inotify_fd,inotify_wd);
bye2:
close(inotify_fd);
return 0;
}
Each of these are compiled and run as system services using a systemd service unit such as:
[Unit]
Description=Atari Kid Grid Hi-scores
After=remote-fs.target
After=syslog.target
# replace /tnfs with your TNFS directory
[Service]
User=thomc
Group=thomc
ExecStart=/usr/local/sbin/kidgrid "/home/thomc/apps.irata.online/Atari_8-bit/Games/High Score Enabled/Kid Grid.atr" "/home/thomc/scores/kidgrid.html"
[Install]
WantedBy=multi-user.target
Since each of these services are written in C, they are not only very small in memory footprint, they have no dependencies other than needing to run on Linux, due to inotify. This can be adjusted to use the notification calls for your favorite operating system:
● kidgrid.service - Atari Kid Grid Hi-scores
Loaded: loaded (/etc/systemd/system/kidgrid.service; enabled; preset: enabled)
Active: active (running) since Mon 2024-12-02 00:03:48 UTC; 1 month 8 days ago
Main PID: 1152 (kidgrid)
Tasks: 1 (limit: 9246)
Memory: 192.0K (peak: 1.5M)
CPU: 5min 53.763s
CGroup: /system.slice/kidgrid.service
└─1152 /usr/local/sbin/kidgrid
Some Games still need help to convert.
The following games are in the atari-game-ports directory, and need help to finish their port.
- Berzerk – Need to find the best place to inject where High Score Table can be triggered.
- moon-patrol-redux – Needs the whole treatment.
- gyruss – Needs a disassembly that is stable and can be patched well.
- star-trek – Needs the whole treatment
- embargo – Can’t get a stable disassembly for #@!(%$
Is there another game that needs to be here? Come help us hack on it!